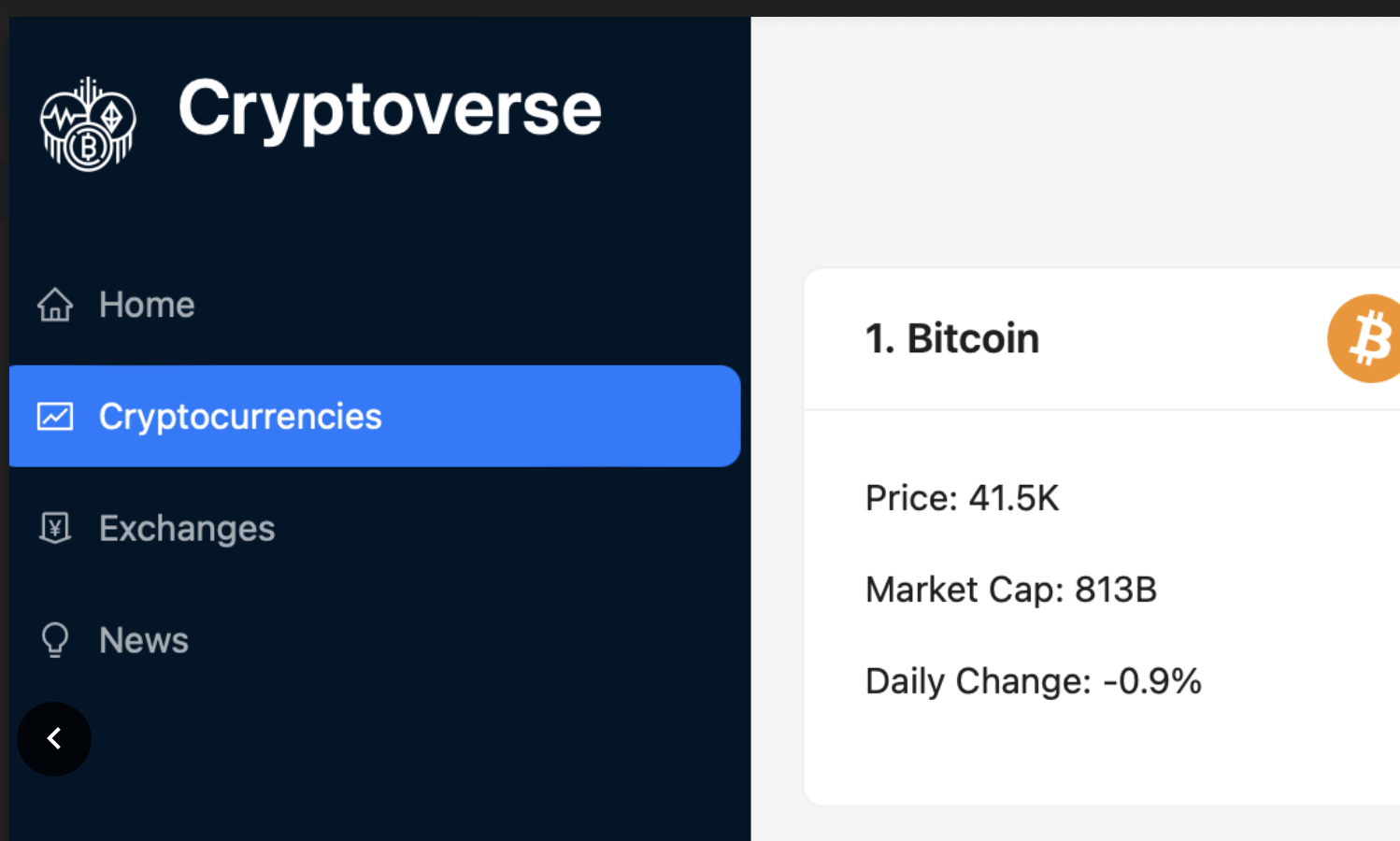
Redux Project
Check out my Redux project using APIs
Click here:
https://franciscoguardadoreduxcryptoapp.netlify.app
Github repo:
https://github.com/franciscojavierguardado101/redux-franciscoguardadoreduxcryptoapp
These are my notes of how I create a Redux appication:
WORKING WITH API = I’D USE RAPID API
npx create-react-app ./
That command initializes an empty react js application in our directory
It will create folders and files
The most important is the src folder
What I like to do is to delete that src folder and then create a new one (of my own)
===
App.js file will be our main functional component
=Then you have to install different dependencies/packages on the so far reactJS project. =
You install designed icons services
Redux/toolbar
Charts.js
And many others so they can be rendered in the app
NPM start to look at my changes
Important:
What is the difference between js files and jsx files:
Essentially they are the same but simply the change on the name “JSX” helps you differentiate which is which between js files and react files
==================
Note:
You need to be more organized when importing icons to let’s say the Navbar or anywhere else
In order to do that you need to create an index.js file on the Components folder and make the imports from there
= for the above explanation:
If you import icons/images to the navbar you would do this, for example:
import { Navbar } from './components';
=Also
The index.js file in the components folder where you actually are more organized when importing you do this:
=
import { default as Navbar } from './Navbar';
Remember to also install following dependency:
NPM install react-router-dom
Run= NPM start
===========================
SRC > INDEX.JS
DO THIS: TO USE LINKS YOU NEED TO WRAP APP CODE IN ROUTER
SRC> COMPONENTS> INDEX.JS
Import all the component files onto the index.js file
Tip for the new guys,
In react-router-dom v6, "Switch" is replaced by routes "Routes". You need to update the import from
import { Switch, Route } from "react-router-dom";
to
import { Routes ,Route } from 'react-router-dom';
You also need to update the Route declaration from
====================================================
Main goal is to fetch all of the real data to the front
Redux toolkit makes writing code much easier
It uses queries
Think about this:
On INDEX.JS we will wrap our entire app inside the Provider and the provider has as variable ‘store’
Store is where we have everything about Redux and will be passing queries
We can import queries from REDUXJS/TOOLKIT
Process:
I created a store in STORE.JS
THEN WE PASS THAT STORE VARIABLE TO PROVIDER THAT WE ARE WRAPPING OUR APP WITH
THEN I CREATED SPECIFIC API TO FETCH DATA FROM =RAPIDAPI
NOW WE NEED TO FIND A WAY TO CONNECT THIS API TO THE STORE:
=THIS IS ACHIEVED BY GOING TO STORE.JS FILE AND MAKE THE IMPORT OF THE API
AND THEN PASS IT THROUGH THE REDUCER
REDUX DOES EVERYTHING FOR YOU
Loading data, when working with redux and APIs you basically have everything you need when you make api calls
=======
TO RENDER COMPONENTS WE HAVE TO IMPORT THEM
useEffect in my component file can help me start filtering which in turn helps with the search button field
The way News section works is that you need Rapid API. You could subscribe to for example Bing News Search and then grab the ‘headers’ from Test Endpoints.
In Redux, once you actually fetch the data and you want to go back and see it, it’s not making another API call. It already has data saved in the cache.
To host it and make a repo to GitHub:
You go to terminal from your root project and use command line:
NPM run build